The Apple App Site Association (AASA) is an important tool for iOS app developers. It securely connects apps to websites and allows apps to use features like universal links and shared web credentials. In today’s digital age, where users move between apps and websites seamlessly, it’s crucial to provide a smooth and secure experience. AASA plays a key role in achieving this goal.
This guide will explore AASA, explaining its mechanisms, benefits, and the step-by-step process of creating and validating your own AASA file. Whether you’re an experienced developer or just starting out, understanding AASA is vital for improving user engagement and ensuring a secure connection between your app and website. The AASA file helps apps collaborate with websites by sitting on a web server and being checked by a user’s device when they install an app.
If everything matches up, the app can work better with the website. Setting up the AASA file is simple. Developers create a JSON file with the necessary information and place it in the correct location on their website so that Apple’s servers can find it. This allows the app and website to work together smoothly.
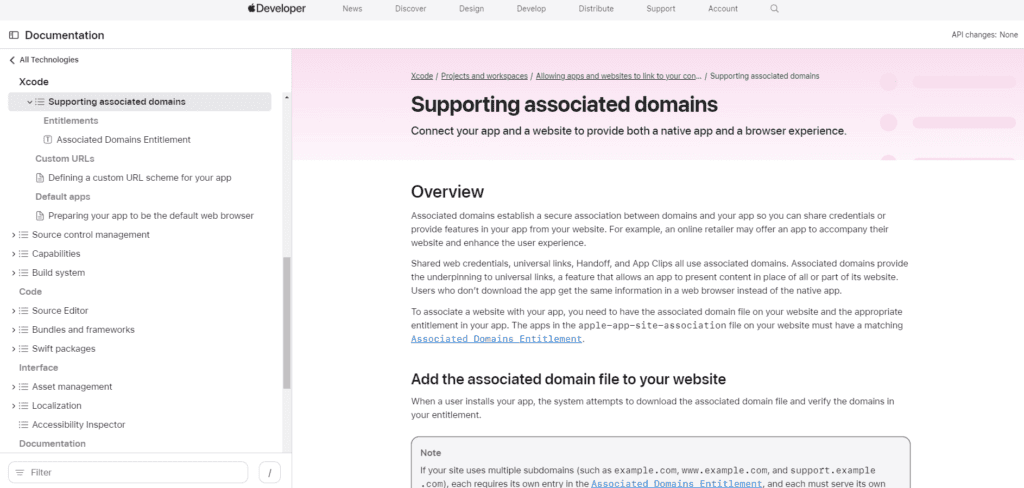
Refer to this page for more documentation from Apple: https://developer.apple.com/documentation/xcode/supporting-associated-domains
Streamlining App and Website Integration
What is Apple App Site Association?
Apple App Site Association (AASA) is a file on your website that establishes a secure connection between your app and website. This connection enables features like Universal Links, Shared Web Credentials, Handoff, and App Clips.
How Does AASA Work?
When a user taps a Universal Link, iOS checks the AASA file on your website to verify if your app can handle the link. If so, the app opens directly; otherwise, the link opens in Safari.
Benefits of Using AASA
- Seamless User Experience: Users can move between your app and website without interruption.
- Enhanced Security: AASA ensures only your app can open links from your website, preventing unauthorized access.
- Improved Discoverability: AASA makes your app content easier to find through web searches and social media.
Creating Your AASA File
- Name your file “apple-app-site-association” (without an extension).
- Use JSON format to structure the file’s content.
- Include details about your app IDs, paths to be handled by your app, and other services you support.
- Place the file in the “.well-known” directory at the root of your website.
AASA File Structure
Key | Description |
---|---|
applinks | Contains information about the app IDs and paths associated with your app. |
apps | An empty array required for Universal Links to function. |
details | An array of dictionaries, each containing information for a specific app ID and its associated paths. |
appID | Your app’s team ID or app ID prefix, followed by the bundle ID. |
paths | An array of strings specifying which parts of your website your app should handle. |
Example AASA File
{
"applinks": {
"apps": [],
"details": [
{
"appID": "9JA89QQLNQ.com.example.app",
"paths": [ "/wwdc/news/", "/videos/wwdc/2015/*" ]
}
]
}
}
Validating Your AASA File
Use the App Search API Validation Tool
provided by Apple to ensure your AASA file is correctly formatted and accessible.
Key Takeaways
- Apple App Site Association links apps and websites
- The file enables features like universal links
- Proper setup improves app and web integration
Implementing Apple App Site Association
Apple App Site Association (AASA) lets iOS apps open web links directly. This section covers creating the AASA file, server setup, and Xcode configuration.
Understanding the AASA File
The AASA file is a JSON file that links your website to your iOS app. It must be named “apple-app-site-association” with no file extension. Place it in your website’s root or in a “.well-known” folder.
The file lists your app IDs and the URLs they can open. Here’s a basic example:
{
"applinks": {
"apps": [],
"details": [
{
"appID": "TEAM_ID.BUNDLE_ID",
"paths": ["*"]
}
]
}
}
Replace TEAM_ID with your Apple Developer Team ID and BUNDLE_ID with your app’s bundle ID. The “paths” array shows which URLs your app can open.
Configuring Your Web Server
Your web server needs to send the AASA file correctly. Set these rules:
- Use HTTPS to serve the file.
- Set the Content-Type to “application/json”.
- Don’t redirect requests for this file.
Many servers use these settings in a .htaccess file:
<Files "apple-app-site-association">
ForceType 'application/json'
</Files>
Test your setup by visiting https://yourdomain.com/apple-app-site-association in a web browser. You should see the JSON content of your file.
Setting Up Associated Domains in Xcode
To use AASA in your app:
- Open your Xcode project.
- Click on your target.
- Go to the “Signing & Capabilities” tab.
- Add the “Associated Domains” capability.
- Add an entry like “applinks.com”.
In your app’s code, handle incoming links. In Swift, use the UIApplicationDelegate method:
func application(_ application: UIApplication,
continue userActivity: NSUserActivity,
restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool {
if let incomingURL = userActivity.webpageURL {
// Handle the URL here
}
return true
}
This setup lets your app open links from your website directly.
Advanced Topics in AASA
The AASA file offers powerful features for iOS app developers. It enables universal links, deep linking, and support for App Clips. These tools can greatly boost user experience and app engagement.
Working with Universal Links and Deep Linking
Universal links let iOS apps handle specific URLs. This creates a smooth link between websites and apps. To set up universal links, add the app’s ID to the AASA file. Include the paths you want the app to handle.
You can use wildcards for more flexible path matching. For example:
{
"applinks": {
"apps": [],
"details": [
{
"appID": "ABCDE12345.com.example.app",
"paths": ["/product/*", "/category/*"]
}
]
}
}
This setup allows the app to open links like “example.com/product/123” or “example.com/category/shoes”.
Deep linking takes users to specific content in your app. You can pass data through URL parameters. Your app then uses this info to show the right screen or content.
Enhancing the User Experience with Shared Web Credentials and Handoff
Shared Web Credentials let apps access saved passwords from Safari. This makes sign-ins easier. To use this, add the “webcredentials” key to your AASA file:
{
"webcredentials": {
"apps": ["ABCDE12345.com.example.app"]
}
}
Handoff lets users start a task on one device and finish on another. It works with websites and apps. To support Handoff, use the “activitycontinuation” key in your AASA file:
{
"activitycontinuation": {
"apps": ["ABCDE12345.com.example.app"]
}
}
These features create a seamless experience across devices and platforms.
Supporting App Clips and Alternate Modes
App Clips are small parts of your app that users can run quickly. They’re great for tasks like placing an order or renting a bike. To support App Clips, add the “appclips” key to your AASA file:
{
"appclips": {
"apps": ["ABCDE12345.com.example.appclip"]
}
}
You can also set up alternate modes for your app. This is useful for things like a “lite” version. Add these modes under the “details” key in your AASA file:
{
"applinks": {
"details": [
{
"appID": "ABCDE12345.com.example.app",
"paths": ["/standard/*"],
"appIDs": ["ABCDE12345.com.example.app.lite"],
"components": [
{
"/": "/lite/*"
}
]
}
]
}
}
This setup lets you handle different paths with different app versions.
Frequently Asked Questions
The Apple App Site Association (AASA) file helps link websites to apps. Here are some common questions about using AASA files.
How can I validate the AASA file for my website?
Apple provides an online tool to check AASA files. Go to the Apple Search Validation Tool website. Enter your domain name. The tool will scan your site and show any issues with the AASA file.
What is the correct path to host the AASA file?
Put the AASA file in the /.well-known/ folder on your web server. The full path should be:
https://example.com/.well-known/apple-app-site-association
Make sure the file is accessible without a file extension.
Can one AASA file support multiple applications?
Yes, a single AASA file can link to many apps. List each app’s details in the “applinks” section of the JSON file. Include the app ID and paths for each app you want to connect.
What steps are involved in setting up deep linking with AASA?
To set up deep linking:
- Create the AASA file with app details and URL paths
- Upload the file to your web server
- Add Associated Domains to your app in Xcode
- Handle incoming URLs in your app code
Test the setup to make sure links open your app correctly.
How often does the AASA file get updated on user devices?
iOS checks for AASA file updates when users open your app. It also checks periodically in the background. The exact timing varies. To push updates faster, users can delete and reinstall your app.
Where can I find comprehensive documentation for AASA implementation?
Apple’s developer website has full details on AASA files. Look for the “Enabling Universal Links” guide in the iOS documentation. It covers file format, hosting, and how to add support in your app.